Qt Slot Signal Tutorial
QtCore.SIGNAL and QtCore.SLOT macros allow Python to interface with Qt signal and slot delivery mechanisms. This is the old way of using signals and slots. The example below uses the well known clicked signal from a QPushButton. The connect method has a non python-friendly syntax. In Qt Designer's signals and slots editing mode, you can connect objects in a form together using Qt's signals and slots mechanism.Both widgets and layouts can be connected via an intuitive connection interface, using the menu of compatible signals and slots provided by Qt Designer.When a form is saved, all connections are preserved so that they will be ready for use when your project is built.
The bonus codes on our Qt Creator Slots And Signals Tutorial site are unique, which means you can use them only if you get to the casino through our website. 18+, T&C Apply, New Customers Only. The issue is that when I start the application (with an email address) it prints out the debug message then nothing happens. I know it is a noob question, but is the signal-slot mechanism works in console mode? Or what else can be the issue?
In the previous parts of this tutorial we built our own custom web browser using PyQt5 widgets. Starting from a basicapp skeleton we've extended it to add support a simple UI, help dialogs and file operations. However, one big feature is missing -- tabbed browsing.
Tabbed browsing was a revolution when it first arrived, but is now an expected feature of web browsers. Being able to keep multipledocuments open in the same window makes it easier to keep track of things as you work. In this tutorial we'll take ourexisting browser application and implement tabbed browsing in it.
This is a little tricky, making use of signal redirection to addadditional data about the current tab to Qt's built-in signals. If you get confused, take a look back at that tutorial.
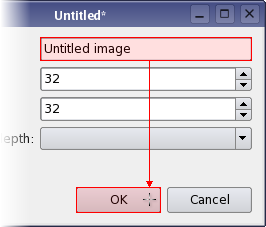
The full source code for Mozzarella Ashbadger is available in the 15 minute apps repository. You can download/clone to get a working copy, then install requirements using:
You can then run Mozzarella Ashbadger with:
Read on for a walkthrough of how to convert the existing browser code to support tabbed browsing.
Creating a QTabWidget
Adding a tabbed interface to our browser is simple using aQTabWidget
. This provides a simple container formultiple widgets (in our case QWebEngineView
widgets)with a built-in tabbed interface for switching between them.
Two customisations we use here are .setDocumentMode(True)
whichprovides a Safari-like interface on Mac, and .setTabsClosable(True)
which allows the user to close the tabs in the application.
We also connect QTabWidget
signals tabBarDoubleClicked
, currentChanged
and tabCloseRequested
to custom slot methods to handle these behaviours.
The three slot methods accept an i
(index) parameter which indicateswhich tab the signal resulted from (in order).
We use a double-click onan empty space in the tab bar (represented by an index of -1
totrigger creation of a new tab. For removing a tab, we use the indexdirectly to remove the widget (and so the tab), with a simple check toensure there are at least 2 tabs — closing the last tab would leave you unable to open a new one.
The current_tab_changed
handler uses a self.tabs.currentWidget()
construct to access the widget (QWebEngineView
browser) of the currently active tab, and then uses this to get the URL of the current page. This same construct is used throughout the source for the tabbed browser, as a simple way to interact with the current browser view.
The code for adding a new tab is is follows:
Signal & Slot changes
While the setup of the QTabWidget
and associated signals is simple,things get a little trickier in the browser slot methods.
Whereas before we had a single QWebEngineView
now there are multipleviews, all with their own signals. If signals for hidden tabs are handled things will get all mixed up. For example, the slot handling aloadCompleted
signal must check that the source view is in a visible tab and only act if it is.
We can do this using a little trick for sending additional data with signals. Below is an example of doing this when creating a new QWebEngineView
in the add_new_tab
function.
As you can see, we set a lambda
as the slot for the urlChanged
signal, accepting the qurl
parameter that is sent by this signal. We add the recently created browser
object to pass into the update_urlbar
function.
Now, whenever the urlChanged
signal fires update_urlbar
will receive both the new URL and the browser it came from. In the slot method we can then check to ensure that the source of the signal matches the currently visible browser — if not, we simply discard the signal.
This same technique is used to handle all other signals which we can receive from web views, and which need to be redirected.This is a good pattern to learn and practice as it gives you a huge amount of flexibility when building PyQt5 applications.
What's next?
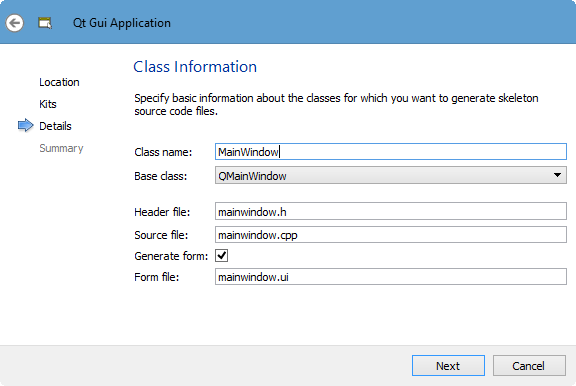
Feel free to continue experimenting with the browser, adding features and tweaking things to your liking. Some ideas you mightwant to consider trying out --
- Add support for Bookmarks/Favorites, either in the menus or as a 'Bookmarks Bar'
- Add a download manager using threads to download in the background and display progress
- Customize how links are opened, see our quick tip on opening links in new windows
- Implement real SSL verification (check the certificate)
Qt Private Slot
Remember that the full source code for Mozzarella Ashbadger is available.
Qt Slot Signal Tutorial Software
Quite a frequent problem when working with signals with slots in Qt5, according to my observations on the forum, is the connection of slots in the syntax on the pointers to signals having an overload of the signature. The same applies to slots that have an overload.
Let's take a test class that has overloaded signals.
Here there is a signal, with an overload of the signature. Connect this signal will also be to the slots that are declared in the Widget class, and which also have an overload of the signature.
How it was in Qt4
Within Qt4, everything was solved quite simply by specifying the signature of the signal and the slot in the SIGNAL and SLOT macros.
How it became in Qt5
Qt Slots Signals Tutorial

But in Qt5, when writing in the new syntax of signals and slots, there are some problems. Because you need to make the static_cast of the method signature.
By the way, the new syntax also allows you to connect signals to slots with a smaller signature, as it was in Qt4.
Advantages of the new syntax
And now a stumbling block. Why use the new syntax of signals and slots? I still hear this question from time to time. Especially when people see such terrible castes of signatures.
- Therefore, I will list potential advantages:The ability to track errors in the connection of signals and slots at the compilation stage, rather than in the runtime
- Reducing compilation time by excluding macros from the code
- The ability to connect lambda functions, it's quite an important bun
- We protect ourselves from errors when we try to connect from the outside to a private slot. Yes!! Yes!! The SIGNAL and SLOT macros ignore the access levels of methods, violating OOP.
In general, for me this is enough, but for you?